What is Space Trail?
Space Trail was created from Katie Noborikawa and Amber Slovic’s love of sci-fi and Oregon Trail. The two dived into creating Oregon Trail even better by raising it up into space. Thus Space Trail was completed.
The crew embarking on this epic quest must fight off thieves, pirates, pesky pigeons, and more to land on their new home.
Encounters in Space
While travelling through space there is the chance of running into dangerous encounters! But that is the risk of travelling through the expanse. Encounters can be space pirates, thieves, weasels, space pigeons, and sicknesses. The encounters vary in danger and losses.
Favorite Functions
While coding Space Trail I created many functions to help clean up code and/or speed up the process. These are my favorite functions used.
//called whenever a crew member gets hurt
function painHappens(){
//chooses a crew member to get hurt
victimRoll = Math.floor((Math.random() * crew.length));
//checks if chosen crew member is dead
if(crew[victimRoll]["status"] == "dead"){
//if they are re roll
painHappens();
} else{
//minuses 2 health from crew member
crew[victimRoll]["health"] -= 2;
if(crew[victimRoll]["health"] <= 0){
crew[victimRoll]["health"] = 0;
}
crewHealth();
}
}
//update the crews health status
function crewHealth(){
totalHealth = 0;
health = 0;
for(var i = 0; i < crew.length; i++){
//calls the reaper if a crew member has 0 health
//but has a status of alive
if(crew[i]['health'] <= 0 &&
crew[i]["status"] == "alive"){
theReaper(i);
}
//adds crew members health to total health
if(crew[i]['status'] == "alive"){
totalHealth += crew[i]["health"];
health++;
}
}
//updates the health on the travel screen
$("span.health").text(health);
}
//the reaper updates the status of crew members
function theReaper(vic){
crew[vic]["status"] = "dead";
c(crew[vic]["name"] + " is dead");
var deadCrewMem;
//counts how many of the crew is dead
for(var i = 0; i < crew.length; i++){
if(crew[i]['status'] == "dead"){
deadCrewMem++;
}
}
//if all are dead then it shows the death screen
if(deadCrewMem >= crew.length){
deadCrew();
}
}
These functions control and update the health of the crew, which essentially determines if you lose the game. The functions are seperated into three peices so that they can be called out of order but linked to the needed function.
function c(print){
console.log(print);
}
Function c was one of the most used functions in my code. The function saved me micro seconds but kept me sound of mind.
function addToConsole(text){
$(".progressConsole").append("" + text + "
");
$(".progressConsole").stop().animate({
scrollTop: $(".progressConsole")[0].scrollHeight
});
}
This function appends text and scrolls the container to the bottom so that the new text is quickly seen. There are two other variations for emphasis, used for landing on planets, and warning, used for alerting player about crew members getting hurt and such.
Color
To keep with Space Trails retro futuristic inspired look the color scheme had to have a neon. The colors also had to be minimal to not detract from the game itself or any of the graphics.
Radioactive
#2DF5D0
Blue Moon
#0B4574
Space Fruit
#FF555D
Yellow Sun
#FFE537
Space
#030D21
Star Light
#EEFFFC
Font
The font choice was inspired by the retro futuristic screens that were dug up in the design research. Using this monospace font created the look of modern analog computer text.
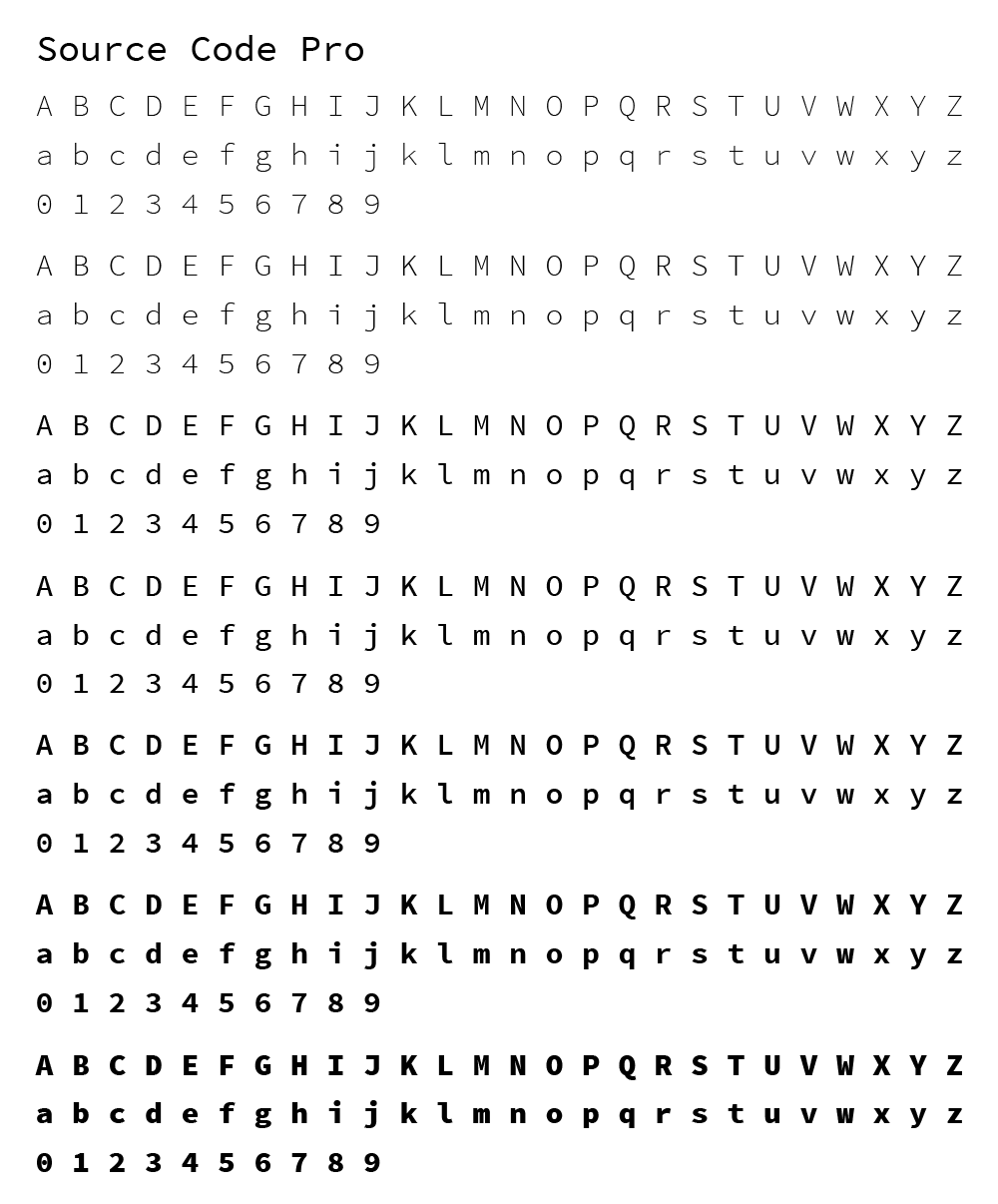